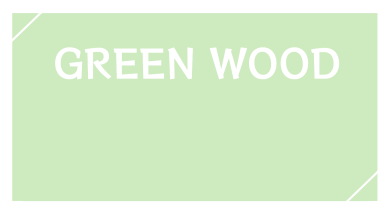
タイトル:クリスマス(12月)
使用言語:p5.js(Javascriptフレームワーク)
プログラミングコード
/*****************
//変数設定
******************/
//手前の雪
let snow;
let snows=[];
//奥の雪
let snow2;
let snows2=[];
//雪だるま
let yukidaruma;
//もみの木
let firtree;
//フォント設定
let font1;
let text1;
let points;
//merry cristmas
let font2;
let text2;
//イルミネーション
let num=20;
let illums=[];
let illum;
//font呼出し
function preload(){
font1=loadFont('fonts/Roboto-Regular.ttf');
font2=loadFont('fonts/AlexBrush-Regular.ttf');
}
function setup(){
//描画するキャンバス設定
//responsible用
if(windowWidth>=820){
cv=createCanvas(970,430,WEBGL);
pg = createGraphics(300,300);
}else if(windowWidth<820 && windowWidth>=450){
cv=createCanvas(windowWidth,430,WEBGL);
pg = createGraphics(300,300);
}else if(windowWidth<450){
cv=createCanvas(windowWidth,200,WEBGL);
pg = createGraphics(200,200);
}
cv.parent('#myCanvas1');
//もみの木インスタンス
firtree=new Firtree();
//テキスト(GREENWOOD)インスタンス
text1=new Text1();
//テキスト(MerryChristmas)
text2=new Text2();
frameRate(15);
}
function draw(){
//背景
background(0,50,50,random(200,220));
//雪奥
if(random(1)>0.5){
snow2=new Snow2();
snows2.push(snow2);
}
for(let i=0;i<snows2.length;i++){
if(!snows2[i].remove()){
snows2[i].move();
snows2[i].show();
}else{
snows2.splice(i,1);
}
}
//もみの木設定
firtree.show();
//テキスト設定(GREENWOOD)
text1.show();
//テキスト設定(Christmas)
text2.show();
//雪だるま
yukidaruma=new Yukidaruma();
//イルミインスタンス
//背後の光彩設定
if(random(1)>0.7){
for(let i=0;i< num;i++){
illum=new Illuminate();
illums.push(illum);
}
}
for(let i=0;i < illums.length;i++){
illums[i].show();
illums.splice(i,1);
}
//手前雪
if(random(1)>0.5){
snow=new Snow();
snows.push(snow);
}
for(let i=0;i < snows.length;i++){
if(!snows[i].remove()){
snows[i].move();
snows[i].show();
}else{
snows.splice(i,1);
}
}
}
/*********************************************
ブラウザ幅を変更したときのキャンバスサイズ再設定
**********************************************/
function windowResized() {
if(windowWidth>=820){
resizeCanvas(970, 520);
}else if(windowWidth < 820){
resizeCanvas(windowWidth, 500);
}
}
/*********************************************
雪だるまクラス
**********************************************/
class Yukidaruma{
//コンストラクタ
constructor(){
//位置とサイズ
if(windowWidth>=820){
this.pos=createVector(-pg.width/2+100,-pg.height/2+70);
this.size=1;
}else if(windowWidth < 820 && windowWidth>=450){
this.pos=createVector(-pg.width/2-80,-pg.height/2+120);
this.size=0.8;
}else if(windowWidth < 450){
this.pos=createVector(-pg.width/2+pg.width/2*1.3,-pg.height/2+pg.height/2);
this.size=0.5;
}
}
//描画メソッド
show(){
push ();
translate (this.pos.x,this.pos.y);
scale(this.size);
pg.textAlign(CENTER, CENTER);
rectMode(CENTER);
pg.textSize(pg.width * 0.8);
pg.text('⛄', pg.width / 2, pg.height / 2);
noStroke();
for (let i = 0; i < width; i +=5) {
for (let j = 0; j < height; j +=5) {
let c = pg.get(i, j);
if(c[0]+c[1]+c[2]>100){
fill(c[0],c[1],c[2],random(100,255));
ellipse(i,j,5);
}
}
}
translate (-this.pos.x,-this.pos.y);
pop ()
}
}
/******************
* テキスト(greenwood)
*******************/
class Text1{
//コンストラクタ
constructor(){
//テキストの配置とサイズ(画面サイズ別)
if(windowWidth>=820){
this.x=-195;
this.y=-60;
this.size=1;
}else if(windowWidth < 820 && windowWidth>=450){
this.x=-148;
this.y=-60;
this.size=1;
}else if(windowWidth < 450){
this.x=-95;
this.y=-20;
this.size=0.5;
}
}
//テキスト描画メソッド
show(){
push();
translate(this.x,this.y);
scale(this.size);
fill (random(255),random(255),0);
//フォントの配列化
points=font1.textToPoints('GREEN WOOD',this.x,this.y,30,
{
sampleFactor:0.5, //dot度 数値大きいほど密になるが表示遅くなる
simplifyThreshold:0
}
);
//フォントの点を描く
for(let i=0;i < points.length;i++){
ellipse(points[i].x,points[i].y,2);
}
translate(-this.x,-this.y);
pop();
}
}
/******************
* テキスト2(Merry Cristmas)
*******************/
class Text2{
//コンストラクタ
constructor(){
//テキストの配置とサイズ(画面サイズ別)
if(windowWidth>=820){
this.x=-50;
this.y=0;
this.size=1;
}else if(windowWidth < 820 && windowWidth>=450){
this.x=-148;
this.y=-60;
this.size=1;
}else if(windowWidth < 450){
this.x=-10;
this.y=10;
this.size=0.4;
}
}
//テキスト描画メソッド
show(){
push();
translate(this.x,this.y);
scale(this.size);
//テキストの設定
textFont(font2);
textSize(80);
fill(200);
text('Merry Cristmas',this.x,this.y)
translate(-this.x,-this.y);
pop();
}
}
/******************
* 雪クラス手前
*******************/
class Snow{
//コンストラクタ
constructor(){
this.pos=createVector(random(-width/2,width/2),random(-height/2,-height/3));
this.vel=createVector(0,1).mult(1.5); //ベクトル設定
this.alpha=random(150,255); //雪の透明度
if(windowWidth>=820){
this.r=random(1,3); //雪のサイズ
}else if(windowWidth < 820 && windowWidth>=450){
this.r=random(1,3); //雪のサイズ
}else if(windowWidth < 450){
this.r=random(0.5,1); //雪のサイズ
}
}
//雪の動きメソッド
move(){
this.pos.add(this.vel);
}
//雪の除去判定メソッド
remove(){
if(this.pos.y>=height){
return true;
}else{
return false;
}
}
//雪の描画メソッド
show(){
push();
translate(this.pos.x,this.pos.y);
scale(this.r);
noStroke();
fill(255,255,255,this.alpha);
ellipse(this.pos.x,this.pos.y,5,5);
translate(-this.pos.x,-this.pos.y);
pop();
}
}
/******************
* 雪クラス奥
*******************/
class Snow2{
//コンストラクタ
constructor(){
this.pos=createVector(random(-width/2,width/2),random(-height/2,-height));
this.vel=createVector(0,1).mult(1.5);
this.alpha=random(30,155); //雪の透明度
if(windowWidth>=820){
this.r=random(1,3); //雪のサイズ
}else if(windowWidth < 820 && windowWidth>=450){
this.r=random(1,3); //雪のサイズ
}else if(windowWidth < 450){
this.r=random(0.5,1); //雪のサイズ
}
}
//雪の動きメソッド
move(){
this.pos.add(this.vel);
}
//雪の除去判定メソッド
remove(){
if(this.pos.y>=height){
return true;
}else{
return false;
}
}
//雪の描画メソッド
show(){
push();
translate(this.pos.x,this.pos.y);
scale(this.r);
noStroke();
fill(255,255,255,this.alpha);
ellipse(this.pos.x,this.pos.y,5,5);
translate(-this.pos.x,-this.pos.y);
pop();
}
}
/******************
* もみのきクラス
*******************/
class Firtree{
constructor(){
//コンストラクタ
if(windowWidth>=820){
this.posx=-300;
this.posy=50;
this.size=0.8;
}else if(windowWidth < 820 && windowWidth>=450){
this.posx=-200;
this.posy=50;
this.size=0.8;
}else if(windowWidth < 450){
this.posx=-100;
this.posy=50;
this.size=0.4;
}
}
//もみの木描画
show(){
push();
translate(this.posx,this.posy);
scale(this.size);
//右半分木の部分
push();
beginShape();
noStroke();
fill(150,100,10);
vertex(0,200);
vertex(50,200);
fill(150,50,10);
vertex(30,50);
vertex(0,50);
endShape();
pop();
//右半分葉の部分
push();
beginShape();
noStroke();
fill(10,115,10);
vertex(0,50);
vertex(200,50);
vertex(100,-50);
vertex(130,-50);
fill(10,random(115,150),10);
vertex(40,-130);
vertex(80,-130);
vertex(0,-200);
endShape();
pop();
push();
beginShape();
noStroke();
fill(150,100,10);
vertex(0,200);
vertex(-50,200);
vertex(-30,50);
vertex(0,50);
endShape();
pop();
push();
beginShape();
noStroke();
fill(10,115,10);
vertex(0,50);
vertex(-200,50);
vertex(-100,-50);
vertex(-130,-50);
fill(10,random(115,150),10);
vertex(-40,-130);
vertex(-80,-130);
vertex(0,-200);
endShape();
pop();
//玉飾り
//左下 紫
push();
fill(sin(frameCount/10)*100,cos(frameCount/10)*100,sin(frameCount/10)*200);
ellipse(-150,20,20,20);
pop();
//左中 青
push();
fill(0,cos(frameCount/10)*100,tan(frameCount/10)*255);
ellipse(-100,-20,20,20);
pop();
//左下 赤
push();
fill(sin(frameCount/10)*255,0,0)
ellipse(50,0,20,20);
pop();
//中 緑
push();
fill(0,cos(frameCount/10)*255,0);
ellipse(-20,-30,20,20);
pop();
//左下 赤
push();
fill(sin(frameCount/10)*200,cos(frameCount/10)*100,sin(frameCount/10)*200);
ellipse(-80,20,20,20);
pop();
//左中上 赤緑
push();
fill(sin(frameCount/10)*255,cos(frameCount/10)*255,0);
ellipse(-50,-80,20,20);
pop();
//中下 黄
push();
fill(sin(frameCount/10)*255,sin(frameCount/10)*255,0);
ellipse(0,20,20,20);
pop();
//中上 緑
push();
fill(sin(frameCount/10)*100,cos(frameCount/10)*255,cos(frameCount/10)*255);
ellipse(0,-150,20,20);
pop();
//中中 青
push();
fill(sin(frameCount/10)*100,cos(frameCount/10)*100,cos(frameCount/10)*255);
ellipse(20,-70,20,20);
pop();
//右中 青
push();
fill(sin(frameCount/10)*100,sin(frameCount/10)*100,cos(frameCount/10)*255);
ellipse(90,-70,20,20);
pop();
//中中
push();
fill(sin(frameCount/10)*100,sin(frameCount/10)*100,cos(frameCount/10)*255);
ellipse(90,-70,20,20);
pop();
//右下
push();
fill(0,sin(frameCount/10)*255,cos(frameCount/10)*255);
ellipse(120,10,20,20);
pop();
//雪
push();
noStroke();
fill(255,255,255,200);
rotate(45);
ellipse(-100,-110,100,30);
pop();
//雪
push();
noStroke();
fill(255,255,255,200);
rotate(45);
ellipse(10,-70,100,30);
pop();
//雪
push();
noStroke();
fill(255,255,255,200);
rotate(-45);
ellipse(-20,-110,100,30);
pop();
translate(-this.posx,-this.posy);
pop();
}
}
/******************
* イルミネーションクラス(背後の光彩))
*******************/
class Illuminate{
constructor(){
this.x=random(-width/2,width/2);
this.y=random(-height/2,height/2);
//背後の光彩サイズ
if(windowWidth>=820){
this.r=random(10,50);
}else if(windowWidth < 820 && windowWidth>=450){
this.r=random(10,50);
}else if(windowWidth < 450){
this.r=random(5,25);
}
}
show(){
push();
beginShape();
translate(this.x,this.y);
noStroke();
fill(random(255),random(255),random(255),random(50,100));
ellipse(this.x,this.y,this.r,this.r);
translate(-this.x,-this.y);
endShape();
pop();
}
}
galleryページへ戻る