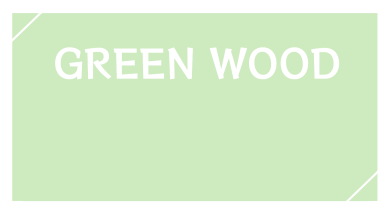
タイトル:節分(2月)
使用言語:p5.js(Javascriptフレームワーク)
プログラミングコード
/*****************
//変数設定
******************/
//手前の雪
let snow;
let snows=[];
//奥の雪
let snow2;
let snows2=[];
//福
let huku;
let hukus=[];
let hukuImg;
//鬼側
let oni;
let oniImg;
//豆側
let mamegawa;
let mameImg;
let beat=[];
/*****************
//画像読み込み
******************/
function preload(){
oniImg=loadImage('oni.png');
mameImg=loadImage('mame.png');
hukuImg=loadImage('huku.png');
}
function setup(){
//描画するキャンバス設定
//responsible用
if(windowWidth>=820){
cv=createCanvas(970,430);
}else if(windowWidth < 820 && windowWidth>=450){
cv=createCanvas(windowWidth,430);
}else if(windowWidth < 450){
cv=createCanvas(windowWidth,200);
}
cv.parent('#myCanvas1');
rectMode(CENTER);
angleMode(DEGREES);
//インスタンス設定
oni=new Oni();
mamegawa=new Mamegawa();
}
function draw(){
//背景
clear ();
background(0,0,0,80);
translate (width/2,height/2);
//雪奥
if(random(1)>0.5){
snow2=new Snow2();
snows2.push(snow2);
}
for(let i=0;i < snows2.length;i++){
if(!snows2[i].remove()){
snows2[i].move();
snows2[i].show();
}else{
snows2.splice(i,1);
}
}
//福
if(random(1)>0.97){
huku=new Huku();
hukus.push(huku);
}
for(let i=0;i < hukus.length;i++){
if(!hukus[i].remove()){
hukus[i].move();
hukus[i].show();
}else{
hukus.splice(i,1);
}
}
//鬼の動き
oni.show();
oni.move();
//豆側の動き
mamegawa.show();
mamegawa.move();
//豆まき(beat)メソッド
for(i=beat.length-1;i>=0;i--){
beat[i].show();
}
//手前雪
if(random(1)>0.5){
snow=new Snow();
snows.push(snow);
}
for(let i=0;i < snows.length;i++){
if(!snows[i].remove()){
snows[i].move();
snows[i].show();
}else{
snows.splice(i,1);
}
}
}
/*********************************************
ブラウザ幅を変更したときのキャンバスサイズ再設定
**********************************************/
// function windowResized() {
// if(windowWidth>=820){
// resizeCanvas(970, 520);
// }else if(windowWidth < 820){
// resizeCanvas(windowWidth, 500);
// }
// }
/******************
* 豆側のクラス
*******************/
class Mamegawa{
constructor(){
if(windowWidth>=820){
//ベクトル設定
this.pos=createVector(0,160);
this.vel=createVector(-1,0).mult(1.5);
//画像を反転させる
this.sizex=1
this.sizey=1;
}else if(windowWidth < 820 && windowWidth>=450){
this.pos=createVector(0,160);
this.vel=createVector(-1,0).mult(1.5);
this.sizex=1
this.sizey=1;
}else if(windowWidth < 450){
this.pos=createVector(0,70);
this.vel=createVector(-1,0).mult(1.5);
this.sizex=0.5
this.sizey=0.5;
}
}
move(){
this.pos.add(this.vel);
if(this.pos.x <= -width/2-300){
this.vel.x=-this.vel.x;
if(windowWidth>=820){
this.sizex=-1
}else if(windowWidth < 820 && windowWidth>=450){
this.sizex=-1
}else if(windowWidth < 450){
this.sizex=-0.5
}
}else if(this.pos.x >= width/2-100){
this.vel.x=-this.vel.x;
if(windowWidth>=820){
this.sizex=1
}else if(windowWidth < 820 && windowWidth>=450){
this.sizex=1
}else if(windowWidth < 450){
this.sizex=0.5
}
}
//Beat用インスタンス作成(ランダムで豆発射)
this.t=random(1);
if(this.t>0.995){
beat.push(new Beat(this.pos.x,this.pos.y,this.vel.x));
}
}
// 四角の描画(端に移動したら反転)
show(){
push();
imageMode(CENTER);
translate(this.pos.x+200,this.pos.y);
rotate(10*sin(30+10*frameCount)); //ふわふわ動かす
scale(this.sizex,this.sizey);
image(mameImg,0,0,100,100);
translate(-this.pos.x-200,-this.pos.y);
pop();
}
}
/******************
* 豆発射クラス
*******************/
class Beat{
constructor(x,y,vxMan){
this.beans=[];
this.num=floor(random(5)); //発射回数
this.vxMan=vxMan; //人の向き(vのプラスマイナス)
print(this.vxMan);
//豆発射インスタンス
//移動方向で発射する角度変更して作成
for(let i=0;i < this.num;i++){
this.beans[i]=[]
if(this.vxMan>0){
for(let j=300;j < 330;j+=10){
this.beans[i][j]=new Bean(x,y,cos(j)*random (4,6),sin(j)*random(4,5));
}
}else{
for(let j=210;j < 240;j+=10){
this.beans[i][j]=new Bean(x,y,cos(j)*random (4,6),sin(j)*random(4.5));
}
}
}
}
//豆発射メソッド
show(){
if(this.vxMan>0){
for(let i=this.num-1;i>=0;i--){
for(let j=300;j < 330;j+=10){
this.beans[i][j].move();
this.beans[i][j].show();
if(this.beans[i][j].remove()){
this.beans.splice(i,1);
}
}
}
}else{
for(let i=this.num-1;i>=0;i--){
for(let j=210;j < 240;j+=10){
this.beans[i][j].move();
this.beans[i][j].show();
if(this.beans[i][j].remove()){
this.beans.splice(i,1);
}
}
}
}
}
}
/******************
* 豆クラス(1粒の豆軌道)
*******************/
class Bean{
constructor(x,y,vx,vy){
//発射位置のベクトル
this.posP=createVector(x,y);
this.velP=createVector(1,0).mult(1.5);
//豆のベクトル
this.pos0=createVector(0,0,0);
this.pos1=createVector(vx,vy);
//速度ベクトル設定
this.vel=p5.Vector.sub(this.pos1,this.pos0).mult(1.5);
this.acc=createVector(0,0.15);
}
//豆の起動
move(){
//発射位置
this.posP.add(this.velP);
//豆のベクトル
this.pos0.add(this.vel);
this.vel.add(this.acc);
}
//豆の配列除去条件
remove(){
if(this.posP.y>height){
return true;
}else{
return false;
}
}
//豆の表示
show(){
translate (this.posP.x+200,this.posP.y);
push ()
translate (this.pos0.x,this.pos0.y);
push ();
fill (255,255,0);
ellipse(this.pos1.x,this.pos1.y,10,10);
pop ();
translate (-this.pos0.x,-this.pos0.y);
pop ()
translate (-this.posP.x-200,-this.posP.y);
}
}
/******************
* 鬼
*******************/
class Oni {
constructor(){
//コンストラクタ
if(windowWidth>=820){
//ベクトル設定
this.pos=createVector(0,150);
this.vel=createVector(-1,0).mult(1.5);
//画像を反転させる
this.sizex=1
this.sizey=1;
}else if(windowWidth < 820 && windowWidth>=450){
this.pos=createVector(0,150);
this.vel=createVector(-1,0).mult(1.5);
this.sizex=1
this.sizey=1;
}else if(windowWidth < 450){
this.pos=createVector(0,70);
this.vel=createVector(-1,0).mult(1.5);
this.sizex=0.5
this.sizey=0.5;
}
}
move(){
this.pos.add(this.vel);
//反転設定
if(this.pos.x===-width/2-100){
this.vel.x=-this.vel.x;
if(windowWidth>=820){
this.sizex=-1
}else if(windowWidth < 820 && windowWidth>=450){
this.sizex=-1
}else if(windowWidth < 450){
this.sizex=-0.5
}
}else if(this.pos.x===width/2+100){
this.vel.x=-this.vel.x;
if(windowWidth>=820){
this.sizex=1
}else if(windowWidth < 820 && windowWidth>=450){
this.sizex=1
}else if(windowWidth < 450){
this.sizex=0.5
}
}
}
show(){
push();
imageMode(CENTER);
translate(this.pos.x,this.pos.y,0);
rotate(10*sin(30+10*frameCount)); //ふわふわ動かす
scale(this.sizex,this.sizey); //向きとサイズ
image(oniImg,0,0,100,100);
translate(-this.pos.x,-this.pos.y,0);
pop();
}
}
/******************
* 福
*******************/
//コンストラクタ
class Huku {
constructor(){
this.pos=createVector(random(-width/2,width/2),-height/2+random(50));
this.vel=createVector(0,1).mult(1.5); //ベクトル設定
this.angle=random(360);
if(windowWidth>=820){
this.size=random(0.5,3);
}else if(windowWidth 6lt 820 && windowWidth>=450){
this.size=random(0.5,3);
}else if(windowWidth < 450){
this.size=random(0.5,1.5);
}
}
move(){
this.pos.add(this.vel);
}
remove(){
if(this.pos.y>=height){
return true;
}else{
return false;
}
}
show(){
push();
imageMode(CENTER);
translate(this.pos.x,this.pos.y,0);
rotate(this.angle+frameCount*5);
scale(this.size);
image(hukuImg,0,0,10,10);
translate(-this.pos.x,-this.pos.y,0);
pop();
}
}
/******************
* 雪クラス手前
*******************/
class Snow{
//コンストラクタ
constructor(){
this.pos=createVector(random(-width/2,width/2),random(-height/2,-height/3));
this.vel=createVector(0,1).mult(1.5); //ベクトル設定
this.alpha=random(150,255); //雪の透明度
if(windowWidth>=820){
this.r=random(1,3); //雪のサイズ
}else if(windowWidth < 820 && windowWidth>=450){
this.r=random(1,3); //雪のサイズ
}else if(windowWidth < 450){
this.r=random(0.5,1); //雪のサイズ
}
}
//雪の動きメソッド
move(){
this.pos.add(this.vel);
}
//雪の除去判定メソッド
remove(){
if(this.pos.y>=height){
return true;
}else{
return false;
}
}
//雪の描画メソッド
show(){
push();
translate(this.pos.x,this.pos.y);
scale(this.r);
noStroke();
fill(255,255,255,this.alpha);
ellipse(this.pos.x,this.pos.y,5,5);
translate(-this.pos.x,-this.pos.y);
pop();
}
}
/******************
* 雪クラス奥
*******************/
class Snow2{
//コンストラクタ
constructor(){
this.pos=createVector(random(-width/2,width/2),random(-height/2,-height));
this.vel=createVector(0,1).mult(1.5);
this.alpha=random(30,155); //雪の透明度
if(windowWidth>=820){
this.r=random(1,3); //雪のサイズ
}else if(windowWidth < 820 && windowWidth>=450){
this.r=random(1,3); //雪のサイズ
}else if(windowWidth < 450){
this.r=random(0.5,1); //雪のサイズ
}
}
//雪の動きメソッド
move(){
this.pos.add(this.vel);
}
//雪の除去判定メソッド
remove(){
if(this.pos.y>=height){
return true;
}else{
return false;
}
}
//雪の描画メソッド
show(){
push();
translate(this.pos.x,this.pos.y);
scale(this.r);
noStroke();
fill(255,255,255,this.alpha);
ellipse(this.pos.x,this.pos.y,5,5);
translate(-this.pos.x,-this.pos.y);
pop();
}
}
galleryページへ戻る