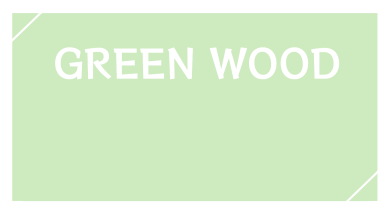
タイトル:バレンタイン(2月)
使用言語:p5.js(Javascriptフレームワーク)
プログラミングコード
/******************
* 変数設定
*******************/
//インスタンス
let pair,choco,heart;
let Num=10;
//キャンバス
let cv,pg,pg2,pg3;
//手前の雪
let snow;
let snows=[];
let hearts=[];
function setup(){
//描画するキャンバス設定
//responsible用
if(windowWidth>=820){
cv=createCanvas(970,430,WEBGL);
pg = createGraphics(300,300);
pg2 = createGraphics(300,300);
}else if(windowWidth < 820 && windowWidth>=450){
cv=createCanvas(970,430,WEBGL);
pg = createGraphics(300,300);
pg2 = createGraphics(300,300);
}else if(windowWidth < 450){
cv=createCanvas(windowWidth,170,WEBGL);
pg = createGraphics(150, 150);
pg2 = createGraphics(150,150);
}
cv.parent("#myCanvas1")
angleMode(DEGREES);
//インスタンス設定
pair=new Pair(); //ペア
choco=new Choco(); //チョコ
}
function draw(){
//画面クリア
clear()
//ペアーメソッド
pair.show();
//チョコメソッド
choco.show();
choco.move();
//ハートメソッド
if(hearts.length < Num){
if(random(1) > 0.98){
for(let i=0;i < Num;i++){
heart=new Heart(cos(i*360/Num),sin(i*360/Num));
hearts.push(heart);
}
}
}
for(let i=0;i < hearts.length;i++){
if(!hearts[i].removal()){
hearts[i].move();
hearts[i].show();
}else{
hearts.splice(i,1);
}
}
//雪メソッド
if(random(1)>0.8){
snow=new Snow();
snows.push(snow);
}
for(let i=0;i < snows.length;i++){
if(!snows[i].remove()){
snows[i].move();
snows[i].show();
}else{
snows.splice(i,1);
}
}
}
/*********************************************
ブラウザ幅を変更したときのキャンバスサイズ再設定
**********************************************/
function windowResized() {
if(windowWidth>=820){
resizeCanvas(970, 430);
}else if(windowWidth < 820){
resizeCanvas(windowWidth, 430);
}
}
/*********************************************
ペアークラス
**********************************************/
class Pair{
//コンストラクタ
constructor(){
this.pos=createVector(-pg.width/2,-pg.height/2+50);
if(windowWidth>=820){
this.size=1;
}else if(windowWidth < 820 && windowWidth>=450){
this.size=1;
}else if(windowWidth < 450){
this.size=0.8;
}
}
//pair
show(){
push ();
translate (this.pos.x,this.pos.y);
scale(this.size);
pg.textAlign(CENTER, CENTER);
rectMode(CENTER);
pg.textSize(pg.width * 0.8);
pg.text('💑', pg.width / 2, pg.height / 2);
noStroke();
for (let i = 0; i < width; i +=8) {
for (let j = 0; j < height; j +=8) {
let c = pg.get(i, j);
if(c[0]+c[1]+c[2]>200){
//fill(random(c[0]),c[1],c[2]);
fill(c[0],c[1],c[2],random(0,255));
ellipse(i,j,8);
}
}
}
translate (-this.pos.x,-this.pos.y);
pop ()
}
}
/*********************************************
チョコクラス
**********************************************/
class Choco{
//コンストラクタ
constructor(){
this.pos=createVector(0,0);
this.vel=createVector(1,0).mult(1.5);
if(windowWidth>=820){
this.size=0.5;
}else if(windowWidth < 820 && windowWidth>=450){
this.size=0.5;
}else if(windowWidth < 450){
this.size=0.3;
}
}
//チョコの動作メソッド
move(){
this.pos.add(this.vel);
if(windowWidth>=820){
this.pos.x=100*cos(frameCount)-100;
this.pos.y=100*sin(frameCount);
}else if(windowWidth < 820 && windowWidth>=450){
this.pos.x=100*cos(frameCount)-100;
this.pos.y=100*sin(frameCount);
}else if(windowWidth < 450){
this.pos.x=50*cos(frameCount)-30;
this.pos.y=50*sin(frameCount)+20;
}
}
//チョコの描画メソッド
show(){
push ();
translate (this.pos.x,this.pos.y);
scale (this.size);
pg2.textAlign(CENTER, CENTER);
rectMode(CENTER);
pg2.textSize(pg2.width * 0.8);
pg2.text('🍫', pg2.width / 2, pg2.height / 2);
noStroke();
for (let i = 0; i < width; i +=10) {
for (let j = 0; j < height; j +=10) {
let c = pg2.get(i, j);
if(c[0]+c[1]+c[2]>100){
fill(random(c[0]),c[1],c[2]);
ellipse(i,j,8);
}
}
}
translate (-this.pos.x,-this.pos.y);
pop ()
}
}
/*********************************************
ハートクラス
**********************************************/
class Heart{
//コンストラクタ
constructor(vx,vy){
this.pos=createVector(random(-width/2,width/2),random(-height/2,height/2));
this.vel=createVector(vx,vy).mult(random(1,2));
this.colR=random(100,555);
this.colG=random(100,555);
this.colB=random(100,555);
if(windowWidth>=820){
this.size=random(3,7);
}else if(windowWidth < 820 && windowWidth>=450){
this.size=random(3,7);
}else if(windowWidth < 450){
this.size=random(1,4);
}
}
//チョコの動作メソッド
move(){
this.pos.add(this.vel);
}
//除去判定
removal(){
if(this.pos.x>width/2 || this.pos.x < -width/2 || this.pos.y>height/2 || this.pos.y<-height/2){
return true;
}else{
return false;
}
}
//チョコの描画メソッド
show(){
push ();
translate (this.pos.x,this.pos.y);
scale (this.size);
colorMode(HSB);
fill(this.colR,this.colG,this.colB,random(0.5,1));
noStroke();
beginShape();
vertex(this.pos.x,this.pos.y);
bezierVertex(this.pos.x - this.size / 2, this.pos.y - this.size / 2, this.pos.x - this.size, this.pos.y + this.size / 3, this.pos.x, this.pos.y + this.size); //ベジェ曲線 描画
vertex(this.pos.x, this.pos.y + this.size);
bezierVertex(this.pos.x + this.size, this.pos.y + this.size / 3, this.pos.x + this.size / 2, this.pos.y - this.size / 2, this.pos.x, this.pos.y); //ベジェ曲線 描画
endShape(CLOSE);
translate (-this.pos.x,-this.pos.y);
pop ()
}
}
/******************
* 雪クラス手前
*******************/
class Snow{
//コンストラクタ
constructor(){
this.pos=createVector(random(-width/2,width/2),random(-height/2,-height/3));
this.vel=createVector(0,1).mult(1.5); //ベクトル設定
this.alpha=random(150,255); //雪の透明度
if(windowWidth>=820){
this.r=random(1,3); //雪のサイズ
}else if(windowWidth < 820 && windowWidth>=450){
this.r=random(1,3); //雪のサイズ
}else if(windowWidth < 450){
this.r=random(0.5,1); //雪のサイズ
}
}
//雪の動きメソッド
move(){
this.pos.add(this.vel);
}
//雪の除去判定メソッド
remove(){
if(this.pos.y>=height){
return true;
}else{
return false;
}
}
//雪の描画メソッド
show(){
push();
translate(this.pos.x,this.pos.y);
scale(this.r);
noStroke();
fill(255,255,255,this.alpha);
ellipse(this.pos.x,this.pos.y,5,5);
translate(-this.pos.x,-this.pos.y);
pop();
}
}
galleryページへ戻る