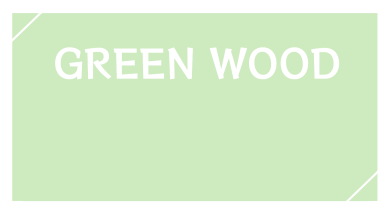
画像をクリックするとハートができます
タイトル:ホワイトデー(3月)
使用言語:p5.js(Javascriptフレームワーク)
プログラミングコード
/******************
* 変数設定
*******************/
//クリック変数
let hearts=[];
//発生させるハート数
const NUM=25;
let hearts2=[];
let deg=0;
function setup(){
//描画するキャンバス設定
//responsible用
if(windowWidth>=820){
cv=createCanvas(970,430,WEBGL);
}else if(windowWidth < 820 && windowWidth>=450){
cv=createCanvas(windowWidth,430,WEBGL);
}else if(windowWidth < 450){
cv=createCanvas(windowWidth,170,WEBGL);
}
cv.parent("#myCanvas1")
angleMode(DEGREES);
colorMode(HSB);
}
function draw(){
//画面クリア
clear()
//クリックハートの発生
for(i=hearts.length-1;i>=0;i--){
hearts[i].updateHeart();
hearts[i].displayHeart();
if(hearts[i].done==true){
hearts.splice(i,1);
}
}
//ハート2(同心円状)の発生位置
let x=random(-width/2,width/2);
let y=random(-height/2,height/2);
//ハート2のインスタンス
if(random(1)>0.98){
for(let i=0;i < NUM;i++){
//ハートを同心円状に生成
p=new Heart2(x,y,cos(i*360/NUM),sin(i*360/NUM),map(i*(360/NUM),0,360,0,300));
hearts2.push(p);
}
}
for(let i=0;i < hearts2.length;i++){
if(!hearts2[i].removal()){
hearts2[i].move(); //ハートの動作メソッド
hearts2[i].show(); //ハートの描画
}else{
hearts2.splice(i,1); //ハート除去
}
}
}
/*********************************************
ブラウザ幅を変更したときのキャンバスサイズ再設定
**********************************************/
function windowResized() {
if(windowWidth>=820){
resizeCanvas(970, 430);
}else if(windowWidth < 820){
resizeCanvas(windowWidth, 430);
}
}
/******************
* クリックイベント
*******************/
//クリックでハートインスタンス生成
function mousePressed(){
hearts.push(new Heart(mouseX,mouseY));
}
/******************
* ハートクラス
*******************/
class Heart{
constructor(x,y){
this.x=x;
this.y=y;
this.r=random(30,50); //ハートサイズ
this.dr=random(1,3); //パーティクル移動量
this.limit=100; //パーティクル寿命
this.fall=false; //崩れ判定
this.done=false; //配列除去判定
this.num=floor(random(30,50));
this.colors=["#abcd5e","#14976b","#2b67af","#62b6de","#f589a3","ef562f","#fc8405","#f9d531"];
this.c=random(this.colors);
//パーティクル生成
this.shape=[];
for(let i=0;i < this.num;i++){
let angle=360/this.num*i;
let pos=this.heartEqn(angle,this.r);
this.shape[i]=new Particle(pos.x,pos.y,this.c);
}
}
updateHeart(){
for(let i=0;i < this.num;i++){
let angle=360/this.num*i;
if(this.fall==false){
//ハートの広がり
this.shape[i].position=this.heartEqn(angle,this.r)
}else{
//ハート崩れる
this.shape[i].updateParticle();
}
}
//崩れるフラグ判定
if(this.r < this.limit){
this.r+=this.dr; //limit内なら広がる
}else{
this.fall=true; //limit以上ならfalse
}
}
//ハート描画
displayHeart(){
push()
//座標中央寄せ
translate(-width/2,-height/2);
translate (this.x,this.y);
let sum=0;
for(let i=0;i < this.num;i++){
this.shape[i].displayParticle();
if(this.shape[i].offScreen()==true){
sum+=1;
}
if(sum==this.num){
this.done=true;
}
}
translate (-this.x,-this.y);
pop ();
}
//ハート関数
heartEqn(angle,r){
//x=√2sin^3(t)
let x=(sqrt(2)*pow (sin(angle),3))*r;
//y=-cos^3(t)-cos^2(t)+2cos(t)
let y=(pow(-cos(angle),3)-pow(cos(angle),2)+2*cos(angle))*-r;
return createVector(x,y);
}
}
/******************
* パーティクルクラス
*******************/
class Particle{
constructor(x,y,c){
this.position=createVector(x,y);
//this.vel=createVector(0,0);
this.vel=p5.Vector.random2D().mult(2);
this.acc=createVector(0,0.1);
this.c=c;
this.d=random(1,10);
}
//パーティクル移動
updateParticle(){
this.vel.add(this.acc);
this.position.add(this.vel);
}
displayParticle(){
noStroke();
fill (this.c);
ellipse(this.position.x,this.position.y,this.d);
}
//パーティクス除去条件
offScreen(){
if(this.position.y>height){
return true;
}else{
return false;
}
}
}
/*********************************************
ハート2クラス
**********************************************/
class Heart2{
//コンストラクタ
constructor(x,y,vx,vy,col){
//始点
this.pos=createVector(x,y);
//速度
this.vel=createVector(vx,vy).mult(2);
this.color=col; //色
if(windowWidth>=820){
this.size=random(30); //ハートのサイズ
this.r=random(50,400); //移動半径
}else if(windowWidth < 820 && windowWidth>=450){
this.size=random(30); //ハートのサイズ
this.r=random(50,400); //移動半径
}else if(windowWidth < 450){
this.size=random(15); //ハートのサイズ
this.r=random(50,200); //移動半径
}
}
//ハートの動作メソッド
move(){
this.pos.add(this.vel);
}
//除去判定メソッド
removal(){
if(this.pos.x>this.r || this.pos.x < -this.r ||this.pos.y>this.r ||this.pos.y < -this.r){
return true;
}else{
return false;
}
}
//ハートの描画
show(){
fill(this.color,100,100,random(0.5,1))
noStroke();
beginShape();
vertex(this.pos.x,this.pos.y);
bezierVertex(this.pos.x - this.size / 2, this.pos.y - this.size / 2, this.pos.x - this.size, this.pos.y + this.size / 3, this.pos.x, this.pos.y + this.size); //ベジェ曲線 描画
bezierVertex(this.pos.x + this.size, this.pos.y + this.size / 3, this.pos.x + this.size / 2, this.pos.y - this.size / 2, this.pos.x, this.pos.y); //ベジェ曲線 描画
endShape(CLOSE);
}
}
galleryページへ戻る