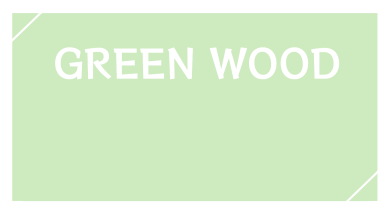
タイトル:こいのぼり(5月)
使用言語:p5.js(Javascriptフレームワーク)
プログラミングコード
/******************
* 変数設定
*******************/
//キャンバス
let cv;
//鯉の数
const num=5;
//こいのぼりの配列設定
let carp;
let carps=[];
//鯉の位置(高さ)上から黒いこいのぼり
let h=[-100,-60,-40,-20,0];
//こいの上半分の色
let col=[[0,0,0],[255,0,0],[0,255,0],[0,0,255],[255,255,0]];
//こいの下半分の色
let col2=[[100,100,100],[255,200,200],[200,255,200],[200,200,255],[255,255,200]];
//サイズ(親→子供)
let size=[0.5,0.4,0.3,0.2,0.1];
//車輪
let wheel;
//泳ぐ鯉用
//泳ぐ鯉の配列設定
let M_carp;
let M_carps=[];
//初期位置
let M_h=[-100,-70,-30,-10,0];
//こいの上半分の色
let M_col=[[0,0,0],[255,0,0],[0,255,0],[0,0,255],[255,255,0]];
//こいの下半分の色
let M_col2=[[100,100,100],[255,200,200],[200,255,200],[200,200,255],[255,255,200]];
//サイズ(親→子供)
let M_size=[0.5,0.4,0.3,0.2,0.1];
//全体移動量
let lx=-250;
let ly=0;
function setup(){
//描画するキャンバス設定
//responsible用
if(windowWidth>=820){
cv=createCanvas(970,430,WEBGL);
}else if(windowWidth<820 && windowWidth>=450){
cv=createCanvas(windowWidth,430,WEBGL);
}else if(windowWidth<450){
cv=createCanvas(windowWidth,170,WEBGL);
}
cv.parent("#myCanvas1")
angleMode(DEGREES);
//鯉のインスタンス
for(let i=0;i<num;i++){
carp=new Carp(0-50*size[i]+lx,h[i]+ly,col[i],col2[i],size[i]);
carps.push(carp);
}
//車輪のインスタンス
wheel=new Wheel();
//泳ぐ鯉のインスタンス
for(let i=0;i<num;i++){
M_carp=new Mcarp(0-50*M_size[i],M_h[i],random(1.5),random(1.5),M_col[i],M_col2[i],M_size[i]);
M_carps.push(M_carp);
}
}
function draw(){
//画面クリア
background(0,0,0,0);
/*****************
//こいのぼりの設定
*****************/
//こいのぼりの設定
for(let i=0;i<carps.length;i++){
carps[i].show(); //こいの描画メソッド
}
//ポールの設定(デバイスサイズごとに変更)
push();
fill(255,200,200);
strokeWeight(3);
//ポールの位置と長さ
if(windowWidth>=820){
line(30+lx,200+ly,30+lx,-150+ly);
}else if(windowWidth<820 && windowWidth>=450){
line(30+lx,200+ly,30+lx,-150+ly);
}else if(windowWidth<450){
line(30+lx,200+ly,30+lx,-100+ly);
}
pop();
//ポールの車輪
wheel.show(); //車輪の描画メソッド
wheel.move(); //車輪の動きメソッド
//泳ぐこいのぼり
for(let i=0;i<M_carps.length;i++){
M_carps[i].show(); //泳ぐこいのぼりの描画メソッド
M_carps[i].move(); //泳ぐこいのぼりの動作メソッド
}
}
/*********************************************
ブラウザ幅を変更したときのキャンバスサイズ再設定
**********************************************/
function windowResized() {
if(windowWidth>=820){
resizeCanvas(970, 430);
}else if(windowWidth<820){
resizeCanvas(windowWidth, 430);
}
}
/****************************/
/*********こいのぼり*********/
/****************************/
//こいのぼりクラス
class Carp{
//コンストラクタ
constructor(x,y,col,col2,size){
this.pos=createVector(x,y);
this.vel=createVector(0,0);
this.col=col; //上半分の色
this.col2=col2; //下半分の色
//こいの配置(画面サイズごとに変更)
if(windowWidth>=820){
this.size=size;
}else if(windowWidth<820 && windowWidth>=450){
line(30+lx,200+ly,30+lx,-150+ly);
this.size=size;
}else if(windowWidth<450){
lx=-150;
ly=50;
this.size=size*0.7;
}
//こいの向き(右方向に向かせる)
this.angle=180;
}
show(){
push();
translate(this.pos.x,this.pos.y);
rotateY(this.angle); //鯉を右に向かせる
fill(this.col);
noStroke();
scale(this.size); //鯉のサイズ設定
//唇部分の描画
push();
stroke(255,255,255);
strokeWeight(5);
line(-100,-50+15*sin(frameCount),-100,50+15*sin(frameCount));
pop();
beginShape();
//鯉の上半分描画
vertex(-100,0);
vertex(-100,-50+15*sin(frameCount));
bezierVertex(-100,-50+random(40,10)*sin(frameCount),0,-100,100,-40);
bezierVertex(100,-40,130,-50,180,-45+random(30,10)*sin(frameCount));
bezierVertex(180,-45,180,10,130,0);
//鯉の下半分描画
fill(this.col2);
vertex(-100,0);
vertex(-100,50+15*sin(frameCount));
bezierVertex(-100,50+random(50,10)*sin(frameCount),0,100,100,40);
bezierVertex(100,40,130,50,180,45+random(30,10)*sin(frameCount));
bezierVertex(180,45,180,-10,130,0);
endShape(close);
//白目描画
beginShape();
fill(255);
ellipse(-50,0+15*sin(frameCount),50);
endShape();
//黒目描画
beginShape();
fill(0);
noStroke();
ellipse(-50,0+15*sin(frameCount),50-20);
endShape();
//鱗の部分描画
beginShape();
noFill();
stroke(150,150,150);
strokeWeight(2);
//arc(x,y,r1,r2,初期角度、終了角度);
arc(0+5*sin(frameCount),-45+5*sin(frameCount),30,30,280,80);
arc(0+5*sin(frameCount),-15+5*sin(frameCount),30,30,280,80);
arc(0+5*sin(frameCount),15+5*sin(frameCount),30,30,280,80);
arc(0+5*sin(frameCount),45+5*sin(frameCount),30,30,280,80);
arc(30+5*sin(frameCount),-28+5*sin(frameCount),30,30,280,80);
arc(30+5*sin(frameCount),0+5*sin(frameCount),30,30,280,80);
arc(30+5*sin(frameCount),28+5*sin(frameCount),30,30,280,80);
arc(60+5*sin(frameCount),-14+5*sin(frameCount),30,30,280,80);
arc(60+5*sin(frameCount),14+5*sin(frameCount),30,30,280,80);
arc(90+5*sin(frameCount),5*sin(frameCount),30,30,280,80);
endShape();
translate(-this.pos.x,-this.pos.y); //これがないとおかしくなる
pop();
}
}
/****************************/
/************車輪************/
/****************************/
class Wheel{
//コンストラクタ
constructor(){
if(windowWidth>=820){
this.x=30+lx; //x座標
this.y=-150+ly; //y座標
this.size=1; //サイズ
}else if(windowWidth<820 && windowWidth>=450){
this.x=30+lx;
this.y=-150+ly;
this.size=1;
}else if(windowWidth<450){
this.x=30+lx;
this.y=-100+ly;
this.size=0.5;
}
//初期角度
this.angle=0;
}
move(){
//車輪を回転
this.angle=this.angle-10;
}
//車輪の描画
show(){
push();
translate(this.x,this.y);
rotate(this.angle);
beginShape();
noFill();
strokeWeight(3);
scale(this.size);
stroke("yellow");
ellipse(0,0,50);
line(35,0,-35,0);
line(0,35,0,-35);
line(35*cos(45),35*sin(45),-35*cos(45),-35*sin(45));
line(-35*cos(45),35*sin(45),35*cos(45),-35*sin(45));
endShape();
translate(-this.x,-this.y);
pop();
}
}
/****************************/
/*********泳ぐこいのぼり*****/
/****************************/
class Mcarp{
//コンストラクタ
constructor(x,y,vx,vy,col,col2,size){
this.pos=createVector(x,y);
this.vel=createVector(vx,vy).mult(0.5);
this.col=col; //鯉の上半身の色
this.col2=col2; //鯉の上半身の色
//こいのサイズ(画面サイズごとに変更)
if(windowWidth>=820){
this.size=size;
}else if(windowWidth<820 && windowWidth>=450){
this.size=size;
}else if(windowWidth<450){
this.size=size*0.5;
}
//こいの向き
this.angle=180;
}
//鯉の動作メソッド
move(){
//左右に接触したら反転
this.pos.add(this.vel);
if(this.pos.x<=-width/2+50 || this.pos.x>=width/2-50){
this.vel.x=(this.vel.x)*(-1);
this.angle=this.angle+180;
}
//上下に接触したら浮上、下降
if(this.pos.y<=-height/2+50 || this.pos.y>=height/2-50){
this.vel.y=(this.vel.y)*(-1);
}
}
//鯉の描画メソッド
show(){
push();
translate(this.pos.x,this.pos.y);
rotateY(this.angle);
fill(this.col);
noStroke();
scale(this.size);
//唇部分
push();
stroke(255,255,255);
strokeWeight(5);
line(-100,-50+15*sin(frameCount),-100,50+15*sin(frameCount));
pop();
beginShape();
//上半分
vertex(-100,0);
vertex(-100,-50+15*sin(frameCount));
bezierVertex(-100,-50+20*sin(frameCount),0,-100,100,-40);
bezierVertex(100,-40,130,-50,180,-45+20*sin(frameCount));
bezierVertex(180,-45,180,10,130,0);
//下半分
fill(this.col2);
vertex(-100,0);
vertex(-100,50+15*sin(frameCount));
bezierVertex(-100,50+15*sin(frameCount),0,100,100,40);
bezierVertex(100,40,130,50,180,45+20*sin(frameCount));
bezierVertex(180,45,180,-10,130,0);
endShape(close);
//白目
beginShape();
fill(255);
ellipse(-50,0+15*sin(frameCount),50);
endShape();
//黒目
beginShape();
fill(0);
noStroke()
ellipse(-50,0+15*sin(frameCount),50-20);
endShape();
//鱗の部分
beginShape();
noFill()
stroke(200,200,200);
strokeWeight(2);
endShape();
beginShape();
arc(0+5*sin(frameCount),-45+5*sin(frameCount),30,30,280,80);
arc(0+5*sin(frameCount),-15+5*sin(frameCount),30,30,280,80);
arc(0+5*sin(frameCount),15+5*sin(frameCount),30,30,280,80);
arc(0+5*sin(frameCount),45+5*sin(frameCount),30,30,280,80);
arc(30+5*sin(frameCount),-28+5*sin(frameCount),30,30,280,80);
arc(30+5*sin(frameCount),0+5*sin(frameCount),30,30,280,80);
arc(30+5*sin(frameCount),28+5*sin(frameCount),30,30,280,80);
arc(60+5*sin(frameCount),-14+5*sin(frameCount),30,30,280,80);
arc(60+5*sin(frameCount),14+5*sin(frameCount),30,30,280,80);
arc(90+5*sin(frameCount),5*sin(frameCount),30,30,280,80);
endShape(close);
translate(-this.pos.x,-this.pos.y);
pop();
}
}
galleryページへ戻る