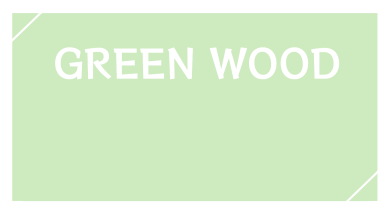
タイトル:花火大会(8月)
使用言語:p5.js(Javascriptフレームワーク)
プログラミングコード
/******************
* 変数設定
*******************/
//花火配列
let fireworks=[];
let font;
/******************
* フォント設定
*******************/
function preload (){
font=loadFont('fonts/Roboto-Regular.ttf');
}
function setup(){
//描画するキャンバス設定
//responsible用
if(windowWidth>=820){
cv=createCanvas(970,430,WEBGL);
}else if(windowWidth < 820 && windowWidth>=450){
cv=createCanvas(windowWidth,430,WEBGL);
}else if(windowWidth < 450){
cv=createCanvas(windowWidth,170,WEBGL);
}
cv.parent('#myCanvas1');
angleMode(DEGREES);
}
function draw(){
//画面クリア
clear ;
background(0,0,0,random(200,250));
//インスタンス
if(random(1) < 0.1){ //数を制限(数が多すぎるので)
fireworks.push(new Firework());
}
//メソッド
for(let i=fireworks.length-1;i>=0;i--){
fireworks[i].explore();
}
}
/********************
* 花火
******************/
class Firework{
constructor(x,y){
this.pos=createVector(random(-width/4,width/4),random(-height/4,height/4)); //位置
this.color=random([[255,0,0],[0,255,0],[100,100,255],[255,255,0],[0,255,255],[255,0,255]]); //色時配列
this.particles=[]; //配列
this.selectflg=random(210); //花火種の選択フラグ
//インスタンス(this.selectflgで花火の種類を変化)
if(this.selectflg < 80){
/*大輪花火*/
this.num=36;
this.r=random([60,70,80]);
for(let i=0;i < 3*this.num+1;i+=1){
if(this.r==60){
//三輪花火
if(i < this.num+1){
this.v=0.5;
}else if(i>this.num && i < 2*this.num){
this.v=1
}else if(i>2*this.num){
this.v=1.5
}
}else if(this.r==70){
//四輪花火
if(i < this.num+1){
this.v=0.5;
}else if(i>this.num && i < 2*this.num){
this.v=1;
}else if(i>2*this.num && i < 3*this.num){
this.v=1.5;
}else if(i>3*this.num){
this.v=2;
}
}else if(this.r==80){
//五輪花火
if(i < this.num+1){
this.v=0.5;
}else if(i>this.num && i < 2*this.num){
this.v=1;
}else if(i>2*this.num && i < 3*this.num){
this.v=1.5;
}else if(i>3*this.num && i < 4*this.num){
this.v=2;
}else if(i>4*this.num){
this.v=2.5;
}
}
let p=new Particle(this.pos.x,this.pos.y,this.v*cos(i*360/this.num),this.v*sin(i*360/this.num),this.r,this.color,0);
this.particles.push(p);
}
}else if(this.selectflg>=80 && this.selectflg < 120){
/*しだれ花火の形状設定*/
this.inid=random(-30,30);
for(let d=60;d < 121;d+=5){
for(let r=1;r < 5;r++){
this.vx=r*cos(d+this.inid);
this.vy=r*sin(d+this.inid);
let p=new Particle(this.pos.x,this.pos.y,this.vx,this.vy,0,this.color,1);
this.particles.push(p);
}
}
}else if(this.selectflg>=120 && this.selectflg < 170){
/*ハート花火の形状設定*/
this.s=5;
this.v=0.1;
this.change=floor(random(5));
for(let angle=0;angle < 361;angle+=10){
this.vx=this.v*((sqrt(2)*pow (sin(angle),3))*this.s);
this.vy=this.v*((pow(-cos(angle),3)-pow(cos(angle),this.change)+2*cos(angle))*-this.s);
let p=new Particle(this.pos.x,this.pos.y,this.vx,this.vy,0,this.color,1);
this.particles.push(p);
}
}else if(this.selectflg>=170 && this.selectflg < 200){
/*フラワー花火の形状設定*/
this.n=floor(random(200));
this.c=0.05;
for(let i=0;i < this.n;i++){
let a=i*137.5;
this.r=this.c*sqrt (i);
this.vx=this.r*cos(a);
this.vy=this.r*sin(a);
this.color=random([[255,0,0],[0,255,0],[100,100,255],[255,255,0],[0,255,255],[255,0,255]]);
let p=new Particle(this.pos.x,this.pos.y,this.vx,this.vy,0,this.color,1);
this.particles.push(p);
}
}else if(this.selectflg>=200){
/*フラワー花火の形状設定*/
this.points=font.textToPoints('GREENWOOD',random(-500,500),random(-height,height),80);
for(let i=0;i < this.points.length;i++){
this.pt=this.points[i];
this.color=random([[255,0,0],[0,255,0],[100,100,255],[255,255,0],[0,255,255],[255,0,255]]);
let p=new Particle(this.pos.x,this.pos.y,this.pt.x*0.01,this.pt.y*0.01,0,this.color,1);
this.particles.push(p);
}
}
}
//描画メソッド
explore(){
push ();
translate (this.pos.x,this.pos.y);
for(let i=this.particles.length-1;i>=0;i--){
this.particles[i].move();
this.particles[i].show();
if(this.particles[i].remove()){
this.particles.splice(i,1);
}
}
translate (-this.pos.x,-this.pos.y);
pop ();
}
}
/********************
* 花火の粒クラス
******************/
class Particle{
constructor(x,y,vx,vy,r,color,shape){
this.pos=createVector(x,y);
//this.vel=p5.Vector.random3D(vx,vy);
this.vel=createVector(vx,vy).mult(1);
this.r=r; //大輪花火半径
this.t=0;
this.lifespan=300; //花火の寿命
this.color=color; //色設定
this.shape=shape; //花火の種類
this.n=random(1);
this.rot=map(this.n,0,1,0,360);
//花火サイズ
if(windowWidth>=820){
this.size=0.5;
}else if(windowWidth < 820 && windowWidth>=450){
this.size=0.4;
}else if(windowWidth < 450){
this.size=0.2;
}
}
//花火の動き
move(){
this.pos.add(this.vel);
this.lifespan -=4; //寿命減少
}
//配列除去条件
remove(){
if(this.lifespan < 0){
return true;
}else{
return false;
}
}
show(){
push ();
translate (this.pos.x,this.pos.y);
scale (this.size);
//落下アクション1
if(this.shape==0){
this.t+=1;
if(this.t>this.r*0.8){
this.vel.x=0;
this.vel.y=4;
rotateX(this.rot)
}
}
//落下アクション2
if(this.shape==1){
this.t+=1;
if(this.t>60){
this.vel.x=random(-2,2);
this.vel.y=2;
rotateY(this.rot)
}
}
//花火の粒
noStroke();
fill (this.color[0],this.color[1],this.color[2],random(255));
ellipse(this.pos.x,this.pos.y,10);
translate (-this.pos.x,-this.pos.y);
pop ();
}
}
galleryページへ戻る